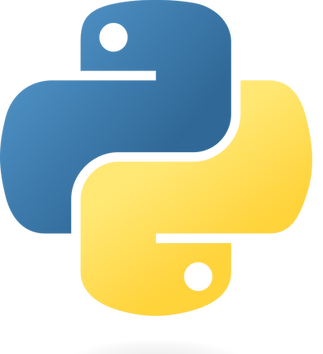
Lesson 1 : Python Basics
In this course, we will explore how to use Python, a widely used programming language in the film industry as well as in various other industries.
​
We will learn how to handle variables, manage conditional structures, work with functions, and many other concepts. Additionally, we will also learn how to use Python in an Integrated Development Environment (IDE).
Table of Contents
1. Introduction to Python
2. First use and first python script
3. Variables and Data Types
4. Basic operations
5. Basic script structure
6. Control structures
7. Conditionals (if, elif, else)
8. Functions
9. Module creation and import
10. Sources
1. Introduction to Python
Python is a versatile programming language, created by Guido van Rossum and first released in 1991. Its design emphasizes code readability and syntactic simplicity, making it an ideal language for both beginners and professionals.
​
Over the years, Python has become one of the most popular
languages in the world, used in a wide range of applications,
from data science and financial analysis to video game
development and system task automation.
​
Throughout the course, you will learn how to create, read, and
understand Python scripts, enabling you to approach programming
projects independently.
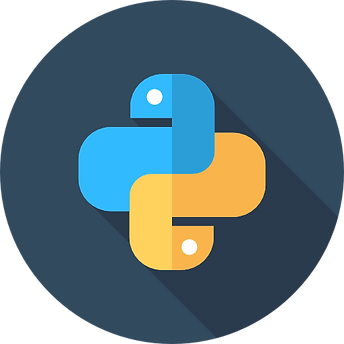
Before you start writing Python scripts, you first need to install Python on your computer. You can download the latest version of Python from the official website by clicking here.
​
The installation process is user-friendly and well-documented.
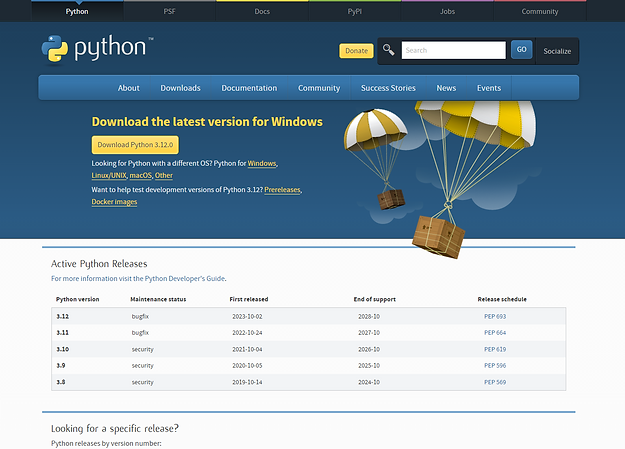
Development Environment
Once Python is installed, to program, we will use an IDE. What is an IDE?
​
In computer programming, an Integrated Development Environment (IDE) is a set of tools designed to enhance the productivity of programmers developing software. It allows for the execution of code and the identification of any potential issues related to our scripts. IDEs offer features like auto-completion, the ability to write and edit multiple lines simultaneously for faster coding.
​
There are several Integrated Development Environments (IDEs) available for Python, including Jupyter Notebook, NotePad++, PyCharm, and VisualStudio.

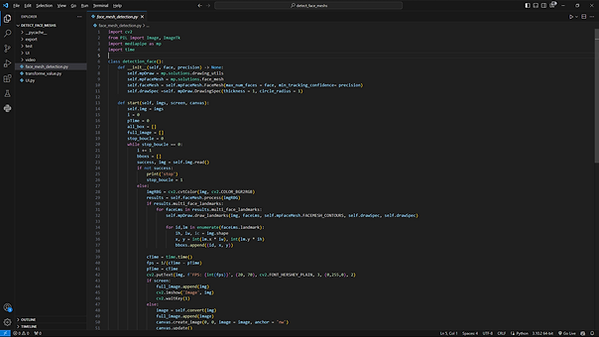

In this course and all others, we will use Visual Studio Code because it provides auto-completion for all the 3D software we will use for scripting and lessons. Additionally, I have a personal attachment to this software because I can seamlessly work with all the programming languages I use.
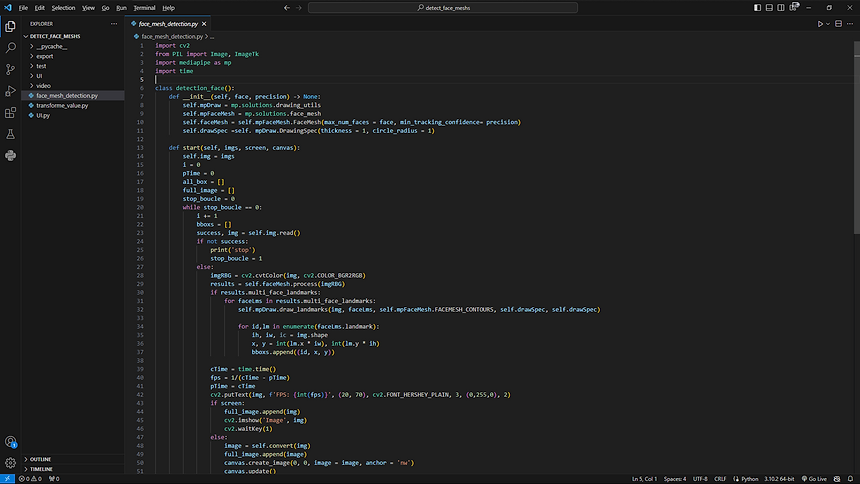
Once Visual Studio Code is installed, you will need to install this extension to be able to run your Python programs with just one click.
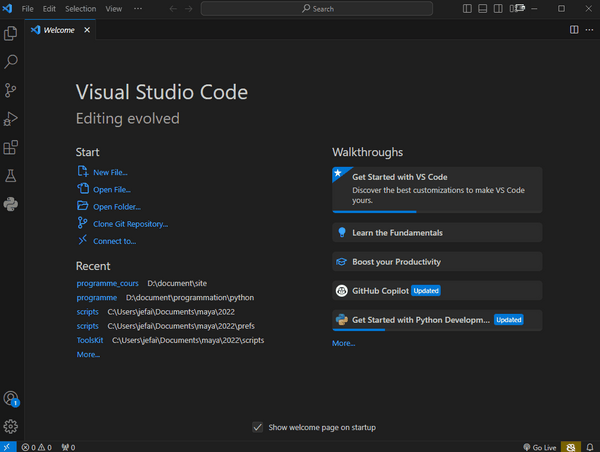
2. First use and first python script
A Python script is simply a text file containing instructions that the Python interpreter can execute. Here are the steps to create your first Python script in Visual Studio:
​
First, you need to create a folder on your computer where you will store all your programs. Then, Open Visual Studio and navigate to the file.
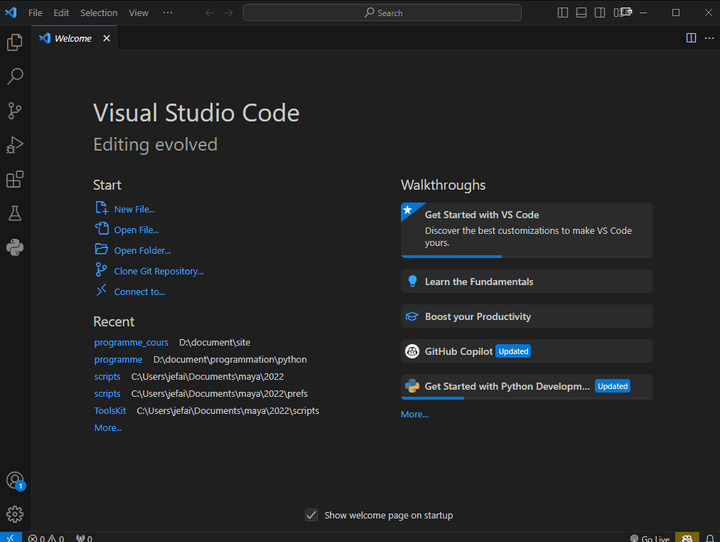
After this, you can write and execute your Python scripts in Visual Studio. To run these Python scripts, you need to press the arrow button, and a terminal window will open at the bottom.
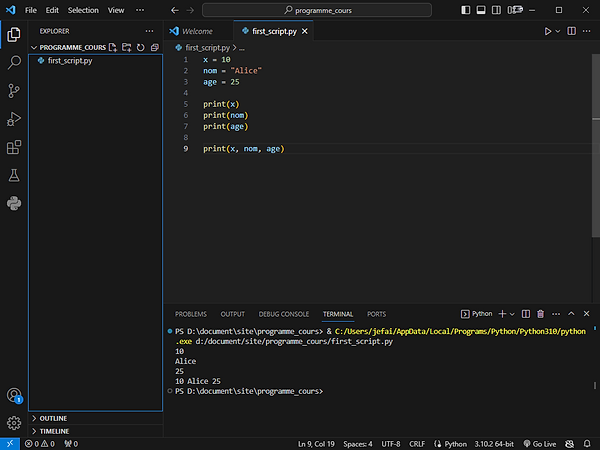
A terminal, or command-line interface, is a user-machine interface where the user interacts with the machine in text mode.
In this case, we use print() which allows us to output various types of variables. print() will display the contents of the variable.
3. Variables and Data Types
​
Variables are containers that allow you to store values. Here's how to define them and assign values in Python:
Variable Declaration:
In Python, you can declare a variable by using a descriptive name (for example, x, name, age) and using the equal sign (=) to assign it a value.
Exemple :
x = 10
nom = "Alice"
age = 25
Certainly! In each example, you can read the variable by adding print().
Example: print(age)
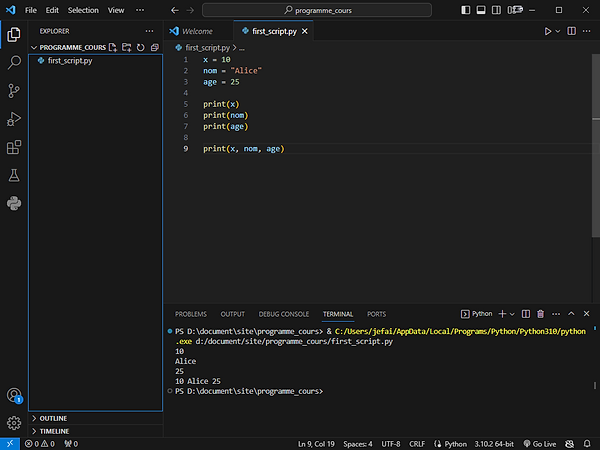
Multiple Assignment: You can assign values to multiple variables at the same time.
Exemple :
a, b, c = 1, 2, 3
In Python, there are several common data types:
Integers (int): They represent whole numbers, both positive or negative.
Floats (float): They represent floating-point numbers.
Strings (str): They represent text and can be enclosed in either single (' ') or double (" ") quotes.
Lists (list): They allow you to store a collection of values, even of different types.
Booleans (bool): They represent truth values (True or False).
Exemple :
variable_int = 42
variable_flottant = 3.14
variable_texte = 'Bonjour'
variable_liste = [ 1, 2, 3, 'quatre', 'cinq' ]
variable_is_true = True
variable_is_false = False
example with visual Studio

4. Basic Operations
Python provides a multitude of operations for manipulating variables and values:
Arithmetic Operations: Addition (+), Subtraction (-), Multiplication (*), Division (/), Modulo (%), Exponentiation (**).
Exemple :
a = 10
b = 3
somme = a + b
difference = a - b
produit = a * b
quotient = a / b
reste = a % b
puissance = a ** b
String Operations: Concatenation (+), Repetition (*).
Exemple :
prenom = "John"
nom = "Doe"
nom_complet = prenom + " " + nom
List Operations: Indexing, Slicing, Concatenation, Adding and Removing Elements.
Exemple :
ma_liste = [1, 2, 3, 4, 5]
premier_element = ma_liste[0]
sous_liste = ma_liste[1:3]
ma_liste.append(6)
ma_liste.remove(4)
5. Basic script structure
A basic Python script has a structure that often includes the following elements:
Imports: At the beginning of the script, you can import external modules that you need for your code.
For example: import math.
Functions: You will usually define functions that contain specific actions your script needs to perform.
Main Code: Apart from the functions, there is often a main code section that calls the functions and performs the main operations of the script.
Comments: Comments (lines preceded by #) are used to explain the code and make the script more readable.
Example of basic script structure:
Exemple :
# Import des modules
import math
# Définition de fonctions
def calcul_carre(nombre):
return nombre ** 2
​
# Code principal
x = 5
resultat = calcul_carre(x)
print(f"Le carré de {x} est {resultat}")
This basic structure is adaptable according to the specific needs of your script.
6. Control structures
Control structures allow you to direct the flow of a program based on certain conditions. They are essential for making decisions and repeating actions. There are primarily two types: for loops and while loops.
​
The for loop allows you to iterate over a given sequence (such as a list, a string, etc.) and execute a block of code for each element in that sequence.
Exemple :
for i in range(5):
print( i )
var_list = ['5', 5, 'yes', 45.85]
for i in var_list:
print( i )
​
The while loop allows you to execute a block of code as long as a condition is true. However, they are rarely used because they can potentially lead to infinite loops, which would indefinitely block your program.
Exemple :
i = 0
while i < 5:
print( i )
i = i + 1
7. Conditionals (if, elif, else)
Conditional structures allow you to execute different blocks of code based on certain conditions.
If Statement: The if structure allows you to execute a block of code if a condition is true. In conditions, you can use the following comparisons:
-
var1 > var2 -- var1 is greater than var2
-
var1 >= var2 -- var1 is greater than or equal to var2
-
var1 == var2 -- var1 is equal to var2
-
var1 != var2 -- var1 is not equal to var2
-
var1 < var2 -- var1 is less than var2
-
var1 <= var2 -- var1 is less than or equal to var2
Exemple :
age = 18
if age >= 18:
print("Vous êtes majeur.")
The elif (else if) structure allows you to test multiple conditions sequentially. If the first condition is false, the program checks the next one. If it is true, it does not check the remaining conditions. This helps optimize the program's execution time. Keep in mind that you need an initial if statement in order to use elif.
Exemple :
note = 75
if note >= 90:
print("A")
elif note >= 80:
print("B")
elif note >= 70:
print("C")
else:
print("D")
The else structure allows you to execute a block of code if none of the previous conditions are true. If the if or elif statements are not executed, the else condition will be executed by default.
Exemple :
age = 15
if age >= 18:
print("Vous êtes majeur.")
else:
print("Vous êtes mineur.")
8. Functions
Functions allow you to encapsulate a reusable block of code. They take parameters as input, perform operations, and can return a result. This helps avoid writing the same lines of code multiple times and can optimize the speed of writing your code.
​
Here is an example to avoid:
Exemple :
var1 = 15
var2 = 5
var3 = 3
var4 = 9
var5 = 2
var6 = 0
resultat1 = var1 + var2
resultat2 = var3 + var4
resultat3 = var5 + var6
print(resultat1)
print(resultat2)
print(resultat3)
This technique should be avoided because we're repeating the same things multiple times. Here, we're repeating the addition and the print statement three times. These are three lines to copy. If it were a more complex calculation, it would be even longer and more tedious to copy.
For this reason, we should use functions. That's the correct approach.
Here's how to define a function in Python:
Exemple :
def my_fonction(parametre1, parametre2):
# Bloc de code
resultat = parametre1 + parametre2
print(resultat)
return resultat
"def" is the keyword used to define a function.
"my_function()" is the function's name.
"parameter1, parameter2" are the parameters that the function takes as input.
"return result" will return the result of the function. This allows you to store the result of the function in a variable, as shown in the following example.
​
To call a function, you simply use its name and provide the desired parameters, like this:
"my_function(15 + 5)".
Exemple :
def my_fonction(parametre1, parametre2):
# Bloc de code
resultat = parametre1 + parametre2
print(resultat)
return resultat
my_fonction( 15 + 5 )
return_of_fonction = my_fonction( 3 + 9 )
my_fonction( 2 + 0 )
print(return_of_fonction)
With this function, "return_of_function = my_function(3 + 9)", the variable "return_of_function" will store the result of the function "my_function(3 + 9)".
Note that if you don't have a 'return' statement in your function, the function will not return any information.
9. Module creation and import
Modules are Python files that contain functions, classes, and other elements that you can use in your scripts. Here's how to create a module:
-
Create a New Python File: Write the functions or elements you want to include in the module.
For example, let's take the function we created above.
-
Save the File: Save it with a descriptive name followed by the .py extension.
Example: my_module.py
Then, to use the functions from this module in another Python script, you can import it.
In this example, we import the module "my_module" and call the function "my_function" that is inside it.
Exemple module create :
def my_fonction(parametre1, parametre2):
# Bloc de code
resultat = parametre1 + parametre2
return resultat
Exemple module create :
import mon_module
resultat = mon_module.my_fonction(10, 20)
print(resultat)
In Python, there are already pre-made modules that you can either download or use directly as they come included with the standard Python library.
For instance, the "math" module allows for more advanced mathematical calculations such as square roots, logarithms, trigonometric functions like cosine and sine, and more.
Exemple :
import math
resultat = math.cos(10)
print(resultat)
Conclusion
We've been able to explore the basics of Python, including variables, conditions, and functions, as well as some of the fundamental tools. Python is a very versatile language that allows you to do practically anything with ease. It will be highly useful for the upcoming courses we'll have on Maya, Nuke, and Houdini.
​
Python's user-friendly nature makes it accessible to anyone, enabling them to create their own programs and scripts, ranging from simple data management to developing their own software or even video games.
​
Now, let's summarize everything we've discussed in a single script using Visual Studio.
Here's a concrete example below:

This Python script performs various operations with variables and uses control structures to make decisions based on certain conditions. Here's a detailed explanation:
Importing the math module:
import math: This imports the math module, which provides additional mathematical functions.
Initializing Variables:
-
number_1 = 10: Initializes the variable number_1 with the integer value 10
-
number_2 = 2.5: Initializes the variable number_2 with the decimal value 2.5
-
listeNumber = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]: Initializes a list called listeNumber with integers from 0 to 9
If-Elif-Else Conditions:
The script evaluates the relationships between number_1 and number_2:
-
If number_1 is equal to number_2, it executes a block of code
-
Otherwise, if it's less, it executes a different block
-
Otherwise, if it's greater, it executes a different block
-
If none of the above conditions are met, it executes the else block
Conditional Code Blocks:
Each conditional code block prints a message corresponding to the condition and performs a mathematical operation.
​
For Loop:
for element in listeNumber: Iterates through each element in the list listeNumber. print(element): Displays each element in the list. number_4 = element + math.sin(number_2): Adds the current element to the sine value of number_2 and stores the result in a new variable number_4.
​
Results:
The results of the mathematical operations are printed in the console.
I hope this lesson has served you well !
10. Sources
https://fr.wikipedia.org/wiki/Python_(langage)
https://code.visualstudio.com/
https://www.autodesk.fr/products/maya/overview?term=1-YEAR&tab=subscription
https://www.autodesk.com/support/technical/article/caas/tsarticles/ts/lC3jaffqnWFyQoLPEPm7n.html
https://docs.python.org/fr/3/tutorial/
Images
https://code.visualstudio.com/
https://www.autodesk.fr/products/maya/overview?term=1-YEAR&tab=subscription